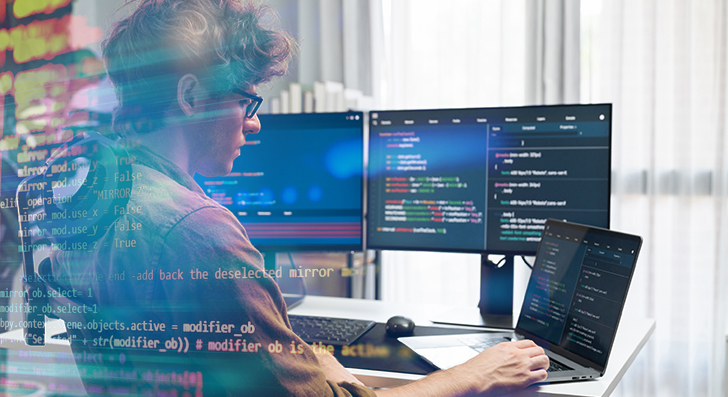
Scalability signifies your software can tackle advancement—far more users, extra knowledge, and a lot more targeted traffic—without having breaking. As a developer, setting up with scalability in mind will save time and stress afterwards. Right here’s a transparent and functional manual that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part of your respective strategy from the start. Numerous apps fall short when they increase fast mainly because the original style and design can’t deal with the additional load. As a developer, you'll want to Assume early about how your program will behave stressed.
Begin by coming up with your architecture to be versatile. Stay clear of monolithic codebases exactly where every thing is tightly linked. In its place, use modular style or microservices. These designs crack your app into more compact, impartial parts. Each and every module or assistance can scale on its own with out influencing The entire program.
Also, take into consideration your database from working day just one. Will it will need to deal with one million people or perhaps a hundred? Pick the ideal style—relational or NoSQL—according to how your knowledge will mature. Approach for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A further vital point is to prevent hardcoding assumptions. Don’t compose code that only performs underneath present-day ailments. Think about what would happen In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use design and style designs that help scaling, like concept queues or occasion-driven methods. These assist your app handle more requests with no acquiring overloaded.
Once you Construct with scalability in mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future head aches. A very well-prepared program is easier to take care of, adapt, and mature. It’s superior to organize early than to rebuild later.
Use the Right Databases
Deciding on the suitable databases is really a key Element of making scalable programs. Not all databases are built a similar, and utilizing the Improper one can gradual you down as well as result in failures as your application grows.
Start off by knowing your data. Could it be extremely structured, like rows inside of a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great suit. They're powerful with interactions, transactions, and consistency. In addition they assistance scaling procedures like browse replicas, indexing, and partitioning to deal with extra targeted traffic and data.
If the information is a lot more flexible—like consumer exercise logs, solution catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured data and may scale horizontally extra effortlessly.
Also, look at your read and publish patterns. Will you be doing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a major create load? Check into databases which can take care of high create throughput, as well as event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Assume ahead. You may not want State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to speed up queries. Stay clear of unnecessary joins. Normalize or denormalize your information based on your accessibility patterns. And often check database efficiency while you expand.
In a nutshell, the ideal databases relies on your application’s framework, pace demands, And the way you count on it to expand. Get time to pick wisely—it’ll conserve lots of difficulty later.
Improve Code and Queries
Fast code is essential to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away anything unwanted. Don’t select the most complicated Alternative if an easy 1 works. Maintain your functions shorter, centered, and easy to check. Use profiling instruments to seek out bottlenecks—locations wherever your code normally takes too very long to run or takes advantage of excessive memory.
Subsequent, check out your database queries. These generally sluggish issues down in excess of the code itself. Be certain Every single question only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative pick precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across massive tables.
For those who recognize the exact same information currently being asked for over and over, use caching. Retail store the results briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in teams. This cuts down on overhead and makes your app a lot more productive.
Make sure to exam with large datasets. Code and queries that function fantastic with one hundred data could crash when they have to handle one million.
Briefly, scalable applications are speedy apps. Keep your code tight, your queries lean, and use caching when required. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of additional buyers plus more traffic. If every thing goes via 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server executing the many operate, the load balancer routes consumers to various servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused swiftly. When consumers request the exact same details once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it through the cache.
There are two prevalent different types of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down database load, enhances pace, and makes your app extra effective.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when info does improve.
In brief, load balancing and caching are uncomplicated but potent instruments. Together, they assist your application deal with additional users, remain rapidly, and Get better from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you require applications that allow your application improve easily. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to buy hardware or guess long term capability. When site visitors will increase, it is possible to insert additional methods with just some clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and security tools. You are able to focus on building your application in lieu of running infrastructure.
Containers are A different essential Device. A container packages your app and all the things it ought to run—code, libraries, settings—into one device. This causes it to be effortless to move your app concerning environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application employs numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's app crashes, it restarts it quickly.
Containers also help it become simple to separate portions of your app into products and services. It is possible to update or scale components independently, and that is great for general performance and dependability.
To put it briefly, employing cloud and container tools suggests you are able to scale rapid, deploy very easily, and Get better promptly when issues materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Observe Every thing
When you don’t monitor your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make greater selections as your application grows. It’s a key Portion of constructing scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application far too. Regulate how much time it's going to take for buyers to load pages, how frequently faults happen, and where they occur. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Set up alerts for crucial difficulties. By way of example, When your response time goes previously mentioned a limit or perhaps website a services goes down, you need to get notified instantly. This helps you fix challenges rapid, generally ahead of consumers even discover.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in glitches or slowdowns, it is possible to roll it back before it will cause true harm.
As your application grows, targeted traffic and information increase. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper applications in position, you continue to be in control.
In short, checking helps you keep the app trusted and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small applications need a powerful Basis. By creating thoroughly, optimizing wisely, and utilizing the ideal resources, you could Develop applications that grow easily devoid of breaking under pressure. Commence little, Consider significant, and Construct clever.